Java for loop is one of the fundamental constructs in the Java programming language, and mastering it is essential for any aspiring developer. In this article, we will explore the intricacies of the Java for loop, its syntax, use cases, and best practices. Understanding how to utilize for loops effectively can greatly enhance your coding skills and optimize your programming efficiency.
By the end of this article, you will be equipped with the necessary skills to implement for loops in your projects seamlessly. So, let’s embark on this journey to mastering the Java for loop!
Table of Contents
- What is a For Loop?
- Syntax of For Loop
- Types of For Loops
- Common Use Cases for For Loops
- Common Pitfalls in Using For Loops
- Performance Considerations
- Best Practices for Using For Loops
- Conclusion
What is a For Loop?
A for loop is a control flow statement that allows code to be executed repeatedly based on a given boolean condition. The for loop is particularly useful for iterating over arrays or collections, making it an essential tool in Java programming.
The for loop consists of three main components: initialization, condition, and increment/decrement. These components work together to control how many times the loop will execute, providing flexibility and efficiency in your code.
Syntax of For Loop
The basic syntax of a for loop in Java is as follows:
for (initialization; condition; increment/decrement) { }
Here’s a brief explanation of each component:
- Initialization: This section is executed once before the loop starts. It is typically used to declare and initialize a loop control variable.
- Condition: This boolean expression is evaluated before each iteration. If it evaluates to true, the loop continues; if false, the loop terminates.
- Increment/Decrement: This part updates the loop control variable at the end of each iteration, allowing control over the number of times the loop runs.
Types of For Loops
Java provides several variations of the for loop, each suited for different scenarios. In this section, we will discuss three primary types of for loops.
Basic For Loop
The basic for loop is the most common type of for loop. It is used for iterating over a range of numbers or through the elements of an array.
for (int i = 0; i < 5; i++) { System.out.println(i); }
In this example, the loop will print the numbers 0 through 4 to the console.
Enhanced For Loop
The enhanced for loop, also known as the for-each loop, was introduced in Java 5 and is used to iterate over arrays and collections without needing an index variable. It simplifies the syntax and improves readability.
int[] numbers = {1, 2, 3, 4, 5}; for (int number : numbers) { System.out.println(number); }
This loop will produce the same output as the previous example but in a more concise manner.
Nested For Loop
A nested for loop is a loop inside another loop. This structure is useful for working with multi-dimensional arrays or when you need to perform multiple iterations.
for (int i = 0; i < 3; i++) { for (int j = 0; j < 2; j++) { System.out.println("i: " + i + ", j: " + j); } }
In this example, the outer loop runs three times, and for each iteration of the outer loop, the inner loop runs two times, resulting in a total of six print statements.
Common Use Cases for For Loops
For loops are versatile and can be used in various scenarios. Here are some common use cases:
- Iterating Over Arrays: For loops are commonly used to process elements in an array.
- Calculating Sums or Averages: You can use for loops to iterate through numbers and perform calculations.
- Generating Patterns: Nested for loops can be used to create patterns or shapes in console output.
- Data Processing: For loops are used to process large datasets efficiently.
Common Pitfalls in Using For Loops
While for loops are powerful, developers can encounter some common pitfalls:
- Off-by-One Errors: This occurs when the loop iterates one time too many or too few. Always double-check your loop conditions.
- Infinite Loops: If the condition never evaluates to false, the loop will run indefinitely, consuming resources.
- Modifying Loop Variables: Changing loop variables within the loop body can lead to unexpected behavior.
Performance Considerations
When using for loops, performance can be a consideration, especially in large-scale applications. Here are some factors to keep in mind:
- Loop Optimization: Modern Java compilers often optimize loops, but writing efficient code is still essential.
- Data Structure Choice: The choice of data structure can impact loop performance. For example, using an ArrayList instead of a LinkedList can lead to better performance in certain scenarios.
- Minimize Computation within Loops: Avoid performing heavy computations inside the loop to enhance performance.
Best Practices for Using For Loops
To maximize the effectiveness of for loops in your Java code, consider these best practices:
- Keep the Loop Body Simple: Ensure that the code inside the loop is straightforward for better readability.
- Use Descriptive Variable Names: This improves code clarity and helps others understand your logic.
- Limit the Scope of Loop Variables: Declare loop variables within the loop to avoid unintended side effects.
- Consider Using Enhanced For Loops: When working with arrays or collections, prefer enhanced for loops for cleaner syntax.
Conclusion
In this comprehensive guide, we have covered the fundamentals of the Java for loop, including its syntax, types, common use cases, and best practices. Mastering the for loop is vital for any Java programmer, as it enhances your ability to write efficient and clean code.
As you continue your journey in Java programming, remember to apply the knowledge gained from this article in your projects. Feel free to share your thoughts in the comments, and don’t hesitate to explore more articles for further learning!
Call to Action
If you found this article helpful, consider sharing it with your peers or leaving a comment below. Additionally, check out our other resources to deepen your understanding of Java and programming concepts.
Final Thoughts
Thank you for reading! We hope to see you back here for more insightful articles on programming and technology. Happy coding!
- Kristy Mcnichol
- La Freeway Protest
- 1534693 Piece Female Characters Deserve Attention
- Tiffany Link Earrings
- Thay Ksada
- Oleksandr Zinchenko
- 1470855 Zack Lugos Biography Age Height Net Worth Girlfriend Brother
- 1230857 Tyler Perry Net Worth Age Height House Wife Son
- Josh Allen Old Tweets

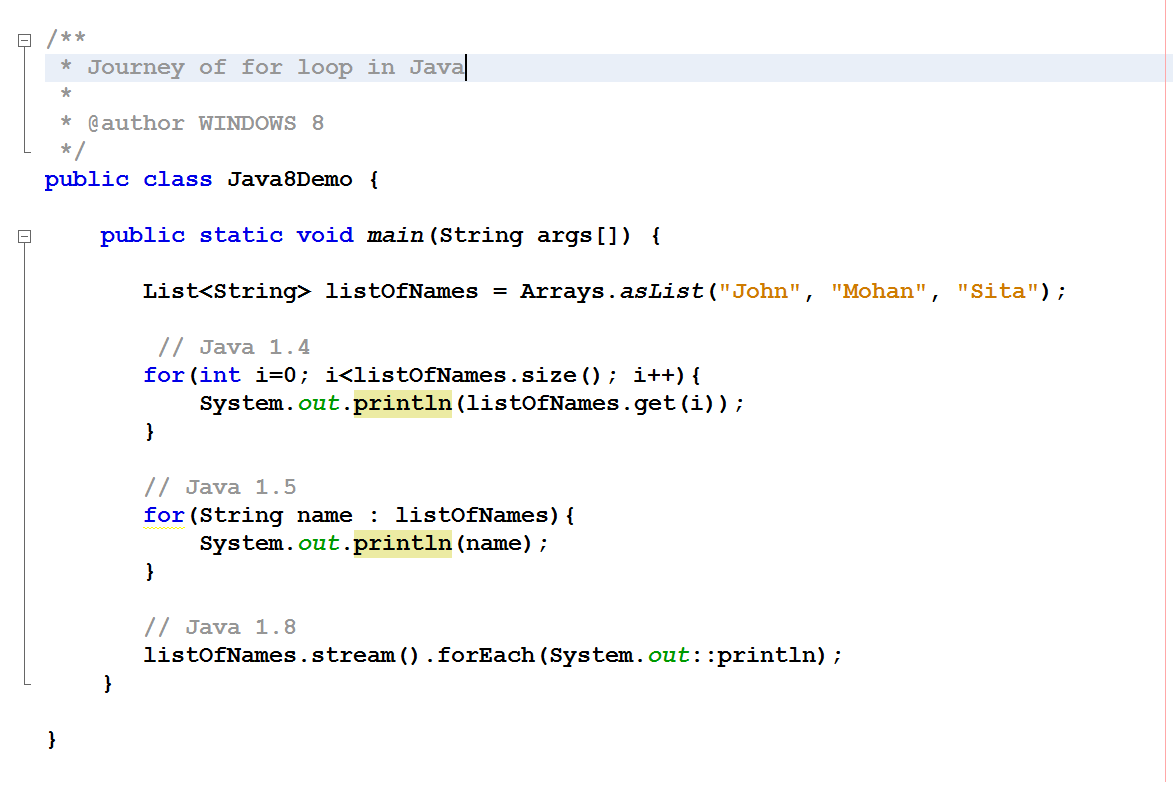
